Share post on
Table of Contents
What is Axios?
Why Set a Base URL in Axios?
When working with APIs in any application, it’s common to send multiple requests to the same API server. Instead of repeating the full API URL every time you make a request, you can use axios set base URL React to define a base URL. This will significantly reduce redundancy and help maintain a cleaner and more organized codebase. You only need to define the relative paths for API endpoints, making your code more modular and scalable.
For instance, instead of writing the full URL in every request, you can create a predefined instance of Axios with a base URL. By using axios set base URL React, it will automatically prepend the base URL to every subsequent request, saving your time and reducing potential errors in typing out long URLs repeatedly.
How to Set Up Axios with a Base URL in React Native
STEP 1 : installing Axios
Before we dive into setting the base URL, you need to install Axios in your React Native project:
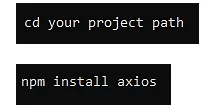
After installation, you can start using Axios to handle your API calls.
STEP 2 : iImporting Axios and Setting up a Base URL
The next step is to import Axios into your React Native project and configure it with a base URL. This will be used for all API requests throughout your app.
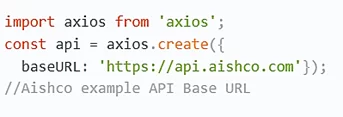
Here, we use axios.create() to create a new instance of Axios and pass in a configuration object that includes the base URL. Now, any requests made with this Axios instance will automatically append the endpoint to the base URL.
STEP 3: Making API Requests
Once you’ve set up the base URL, you can start making requests without having to specify the full URL every time. Here’s an example of how to make GET and POST requests:
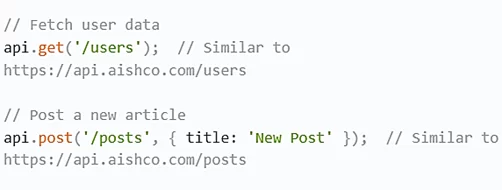
Notice that you only need to pass the relative URL, such as /users or /posts, and Axios automatically appends it to the base URL.
Main API Request Types
Now that you know how to set a base URL, let’s go over the different types of requests you can make with Axios.
GET Request | POST Request |
---|---|
Fetch data from the server | Send new data to the server |
api.get('/data')
|
api.post('/data', { name: 'aishcosolutions' })
|
PUT Request | DELETE Request |
---|---|
Update existing data on the server | Remove data from the server |
api.put('/data/1', { name: 'consultancyashco' })
|
api.delete('/data/1')
|
Error Handling |
---|
Handle errors from the server |
api.get('/data')
|
Handling errors is crucial for creating a seamless user experience. With Axios, error handling is simplified with the .catch() block. You can log the error or show a user-friendly message to indicate that something went wrong.
Key Features of Axios for Efficient API Request Management in React Native
Feature | Description | Benefit |
---|---|---|
Base URL Setup | Define a base URL for all API requests. | Reduces redundancy and keeps code cleaner and more maintainable. |
Interceptors | Modify requests or responses before they are handled. | Simplifies tasks like token management and global error handling. |
Promise-based Handling | Uses promises for asynchronous operations. | Makes API calls easier to manage with .then() and .catch() . |
Timeout Handling | Set a timeout for requests. | Prevents the app from hanging on long-running or failed requests. |
Error Handling | Simplified error management using .catch() . |
Enhances user experience by displaying proper error messages. |
Request Cancellation | Cancel requests if not needed anymore (e.g., when navigating away). | Optimizes performance and reduces unnecessary network usage. |
Browser Compatibility | Works across both browser and mobile environments. | Ensures consistency in code for React and React Native apps. |
Token Management | Attaches tokens to headers automatically via interceptors. | Improves security and reduces code duplication for authentication. |
Advantages of Using Axios in React Native
Here are some advantages of using Axios to manage API requests in React Native:
Base URL Setup
By setting a base URL, you avoid redundancy and keep your code organized.
Interceptors
Axios allows you to intercept requests and responses before they are handled, making it easier to manage tokens or handle errors globally.
Promise-Based
Axios is based on promises, making it easier to work with asynchronous JavaScript and manage API calls with .then() and .catch().
Timeout Handling
You can easily set timeouts for requests to avoid hanging or long-running requests.
Managing API Requests Efficiently with Axios
Best Practices for Using Axios in React Native
To ensure your API requests are efficient and your app remains responsive, follow these best practices:
Set a Base URL
Always define a base URL for your Axios instance, as demonstrated earlier. This will make your code cleaner and easier to maintain.
Use Interceptors
Interceptors can help you modify requests before they are sent or responses before they are processed, which is useful for tasks like adding authorization headers.
Handle Errors Gracefully
Make sure to include proper error handling for all API requests, ensuring that your app remains functional even when API calls fail.
Optimize Requests
If possible, batch multiple requests together or reduce the frequency of requests to avoid overwhelming your API or causing unnecessary network load.
Enhancing Performance with Axios in React Native
Request Throttling
Implement throttling to limit the rate of API requests, improving performance and reducing server load.
Batch Requests
Combine multiple API requests into a single call to reduce network overhead and improve efficiency.
Lazy Loading
Use lazy loading techniques to fetch data only when needed, enhancing initial load times and user experience.
Simplifying Token Management with Axios
In applications that require user authentication, managing tokens for API requests is crucial. By using axios set base URL React, you can streamline your API calls, reducing repetitive code and making it easier to manage authentication across your app. Axios also makes it easy to attach authorization tokens to your requests using interceptors.
By setting up an interceptor, along with axios set base URL React, you can automatically add the token to the headers of every request, ensuring secure communication with your API without repeating the same logic. This approach enhances security and reduces the chances of human error when managing authentication in your React Native app.
Resources for Mastering Axios in React Native
Axios Documentation
Explore the official Axios documentation for a comprehensive guide on its features and usage. Axios GitHub Repository.
React Native Documentation
Learn more about integrating Axios into your React Native project from the official React Native documentation. React Native Docs.
Axios Best Practices
Read about best practices and advanced configurations for using Axios in your applications. Axios Best Practices.
Handling API Requests with Redux
Conclusion
Transform Your Front-End Development with Axios and React Native
Our company specializes in custom web applications, providing solutions using the latest technologies. It develops innovative mobile apps for both iOS and Android platforms to engage users effectively. Expert business consulting services are available to enhance operations through modern technologies.
In e-commerce, the company builds platforms to improve customer experience and boost online sales. Additionally, staff augmentation services offer skilled developers to support projects. Our digital marketing strategies focus on enhancing online presence and reaching target audiences across various applications.
Share post on